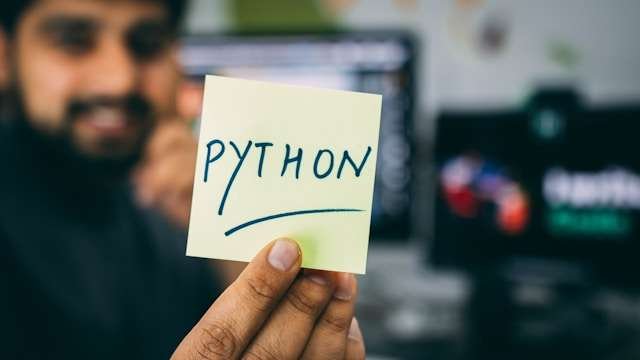
In this article we are briefly going to show how to generate random numbers with python using the standard library.
For more information you can check this page https://docs.python.org/3/library/random.html#random, and the ending point will be something like the code below
from random import seed
from random import random
seed(1)
counter = 0
while counter < 10:
print(random())
counter += 1
and this is the output
0.13436424411240122
0.8474337369372327
0.763774618976614
0.2550690257394217
0.49543508709194095
0.4494910647887381
0.651592972722763
0.7887233511355132
0.0938595867742349
0.02834747652200631
Generate Random Integers
For generating random integers we need to import the randint function that takes 2 arguments (min, max) and will generate a random number between min and max (included)
from random import seed, randint
seed(1)
print(randint(0,10))
Generate Random Floats
For generating random float numbers we can just use random() that will generate a random number between 0 and 1
from random import seed, random
seed(3)
print(random())
Hope this will help
Share this content: