
In this article, we’ll guide you through a few simple steps to bootstrap your Laravel application on Docker, enabling you to set up your entire development environment in just a few minutes.
What is Laravel
In the vast landscape of web development frameworks, Laravel stands out as a beacon of modernity, simplicity, and efficiency. Its rise to prominence has revolutionized the way developers build web applications, offering a rich ecosystem of tools and features that streamline development workflows and empower developers to create robust and scalable applications with ease. In this article, we’ll delve into what Laravel is, why it’s important to know, and the myriad benefits it brings to the table.
What is Laravel? Laravel is an open-source PHP framework that provides an elegant and expressive syntax for writing code. It follows the Model-View-Controller (MVC) architectural pattern, which separates the application logic into three interconnected components: models, views, and controllers. Laravel’s philosophy revolves around simplicity, clarity, and developer happiness, making it a preferred choice for building modern web applications.
More information on Laravel can be found here https://laravel.com/
Before we move next we have to spend few words on PHP Composer
PHP Package manger: Composer
As per each modern language, also PHP has a package and dependency manager. This tool is called Composer. PHP Composer is a dependency manager for PHP that simplifies the process of managing and installing PHP packages and libraries. It allows developers to declare the libraries and dependencies their projects require and then automatically installs and manages them, ensuring that the project’s dependencies are always up-to-date and compatible.
Composer Key Concepts:
- Dependency Management:
- Composer manages dependencies at the project level by creating a
composer.json
file that specifies the required packages and their versions. This file acts as a manifest for the project’s dependencies and defines the project’s dependencies, including the package name, version constraints, and other metadata.
- Composer manages dependencies at the project level by creating a
- Package Repository:
- Composer relies on Packagist, the default package repository for PHP, to discover, download, and install packages. Packagist hosts thousands of PHP packages and libraries contributed by the PHP community, making it easy for developers to find and use existing solutions in their projects.
- Autoloading:
- Composer generates an autoloader file (
autoload.php
) that autoloads classes and files from the installed packages, eliminating the need for manualrequire
orinclude
statements. This autoloader follows the PSR-4 (PHP Standards Recommendation) autoloading standard, allowing developers to organize their code in a predictable and consistent manner.
- Composer generates an autoloader file (
- Dependency Resolution:
- Composer resolves dependencies by analyzing the
composer.json
file and resolving version constraints specified for each package. It ensures that all dependencies are compatible and resolves any conflicts between package versions by selecting the most suitable versions that satisfy all requirements.
- Composer resolves dependencies by analyzing the
- Dependency Installation:
- Composer installs dependencies by executing the
composer install
command, which reads thecomposer.json
file, resolves dependencies, and downloads the required packages into thevendor
directory of the project. Composer also generates acomposer.lock
file that locks the versions of the installed packages, ensuring consistent dependency resolution across different environments.
- Composer installs dependencies by executing the
- Dependency Update:
- Composer allows developers to update dependencies using the
composer update
command, which reads thecomposer.json
file, checks for available updates for installed packages, and updates them to the latest compatible versions. Developers can specify version constraints in thecomposer.json
file to control which versions of packages are allowed to be updated.
- Composer allows developers to update dependencies using the
Why is Composer Important:
- Dependency Management:
- Composer simplifies the process of managing project dependencies by automating package installation, version resolution, and dependency management. It reduces manual effort and ensures that projects have all the required dependencies to function properly.
- Code Reusability:
- Composer enables developers to leverage existing PHP packages and libraries contributed by the PHP community. By using Composer to install third-party packages, developers can reuse code, accelerate development, and focus on building unique features instead of reinventing the wheel.
- Dependency Versioning:
- Composer provides fine-grained control over package versions and dependencies through version constraints specified in the
composer.json
file. This allows developers to specify exact version requirements or use version ranges to ensure compatibility while staying up-to-date with the latest package releases.
- Composer provides fine-grained control over package versions and dependencies through version constraints specified in the
- Standardized Autoloading:
- Composer’s autoloading mechanism follows the PSR-4 autoloading standard, providing a standardized way to autoload classes and files from installed packages. This promotes code organization, modularity, and interoperability between different PHP projects and libraries.
- Community Collaboration:
- Composer fosters collaboration and knowledge sharing within the PHP community by providing a centralized package repository (Packagist) where developers can publish, discover, and share their PHP packages and libraries. This encourages community contributions, code sharing, and ecosystem growth.
In summary, Composer is a valuable tool for PHP developers, enabling them to manage dependencies, leverage existing libraries, and streamline the development process. By embracing Composer, developers can build scalable, maintainable, and interoperable PHP applications while benefiting from the vibrant PHP ecosystem and community contributions. More information on Composer and how can be installed can be found here https://getcomposer.org/download/
What is MVC and why is important
MVC, which stands for Model-View-Controller, is a software architectural pattern used in web development to separate the application’s logic into three interconnected components: the Model, the View, and the Controller. Each component serves a distinct role in managing the application’s data, presentation, and user interactions. Let’s delve into each component and understand why MVC is important:
- Model:
- The Model represents the application’s data and business logic. It encapsulates data manipulation, validation, and storage operations. Models interact with the database, perform CRUD (Create, Read, Update, Delete) operations, and enforce business rules. In essence, the Model acts as the gateway to the application’s underlying data, providing an abstraction layer that shields the rest of the application from the complexities of data storage and retrieval.
- Importance: Separating data-related logic into a dedicated component enhances code organization, reusability, and maintainability. It allows developers to focus on implementing business rules and data manipulation without coupling these concerns with presentation or user interaction logic.
- View:
- The View represents the presentation layer of the application. It is responsible for rendering the user interface and presenting data to the user in a visually appealing and interactive manner. Views typically consist of HTML, CSS, JavaScript, and template markup. They receive data from the Controller or directly from the Model and display it to the user. Views are passive components that do not contain business logic but rather focus on presenting data and responding to user input.
- Importance: Separating the presentation layer from the rest of the application logic promotes code modularity, flexibility, and maintainability. It enables developers to change the look and feel of the application without affecting its underlying functionality. Additionally, separating presentation concerns from business logic facilitates parallel development, allowing front-end and back-end developers to work independently on their respective components.
- Controller:
- The Controller acts as an intermediary between the Model and the View. It receives user input, processes requests, and coordinates interactions between the Model and the View. Controllers contain application logic related to handling user requests, routing requests to the appropriate actions, invoking methods on the Model to retrieve or manipulate data, and passing data to the View for rendering. Controllers encapsulate the application’s flow and logic, orchestrating the interaction between the user, the data, and the presentation layer.
- Importance: Separating request handling and application logic from presentation concerns promotes code reusability, testability, and scalability. Controllers decouple user interactions from data manipulation, allowing developers to write modular, maintainable, and testable code. Moreover, separating concerns into distinct components enhances code readability and makes it easier to understand and debug complex applications.
MVC Diagram
Now, let’s visualize how these components interact, see the diagram below for further reference.
- The browser/User sends a request to the Controller.
- The Controller interacts with the Model to send and receive data (fetching or updates database record).
- The Controller also communicates with the View to update what the user sees (displaying the selected database record).

Why is Laravel Important to Know?
- Rapid Development: Laravel offers a suite of pre-built components and libraries that accelerate the development process. From authentication and authorization to database migrations and routing, Laravel simplifies common tasks, allowing developers to focus on building unique features and functionalities.
- Modern Tooling: Laravel embraces modern PHP features and best practices, such as dependency injection, service providers, and artisan command-line interface. These tools enhance code maintainability, testability, and scalability, ensuring that Laravel applications remain robust and future-proof.
- Community Support: Laravel boasts a vibrant and active community of developers who contribute plugins, packages, and resources to the Laravel ecosystem. The community provides documentation, tutorials, forums, and online resources that facilitate learning, troubleshooting, and collaboration.
- Built-in Security: Security is a top priority in Laravel, with features such as CSRF (Cross-Site Request Forgery) protection, XSS (Cross-Site Scripting) filtering, input validation, and encryption out of the box. Laravel’s security measures mitigate common web security threats, safeguarding applications and user data.
- Scalability and Performance: Laravel is designed with scalability and performance in mind, featuring tools like Eloquent ORM (Object-Relational Mapping), database migrations, and caching mechanisms that optimize application performance and scalability. Laravel applications can handle high traffic loads and scale seamlessly as they grow.
Benefits of Using Laravel
- Elegant Syntax: Laravel’s clean and expressive syntax simplifies code readability and comprehension, reducing cognitive overhead and making code maintenance a breeze.
- Powerful ORM: Laravel’s Eloquent ORM provides an intuitive and fluent interface for interacting with databases, enabling developers to define database relationships, perform CRUD (Create, Read, Update, Delete) operations, and execute complex queries with ease.
- Blade Templating Engine: Laravel’s Blade templating engine offers a powerful yet simple syntax for writing dynamic and reusable templates. Blade templates support features like template inheritance, control structures, and includes, enhancing code organization and maintainability.
- Artisan CLI: Laravel’s Artisan command-line interface automates common development tasks such as generating code scaffolds, running database migrations, seeding databases with test data, and managing application assets. Artisan commands boost developer productivity and reduce manual labor.
- Laravel Ecosystem: Laravel’s rich ecosystem encompasses a wide range of official and community-driven packages, integrations, and extensions that extend Laravel’s functionality. From authentication and authorization to caching and queueing, Laravel packages provide solutions to common development challenges, saving time and effort.
How to Bootstrap your Laravel application on Docker
For bootstrapping our application in few minutes all we need is:
- docker-engine
- docker-compose
- composer
- git client
Step 1: Download the code repo
git clone https://github.com/brewedbrilliance/laravel-initial
Navigate into the laravel-initial folder
cd laravel-initial
Step 2: Build the environment using the init.sh script
sh init.sh my_local_laravel_dev -f
This script will check that all the dependencies are met (docker, docker-compose), will build the container images (mysql and php/apache) and will then wait to be fully up so that can run the php composer script for installing the laravel application and running the database migrations.
In a short amount of time (few mins or less) you will have running on docker your laravel dev environment

That can be accessed through the browser by reaching
http://localhost:8081/my_local_laravel_dev/public/
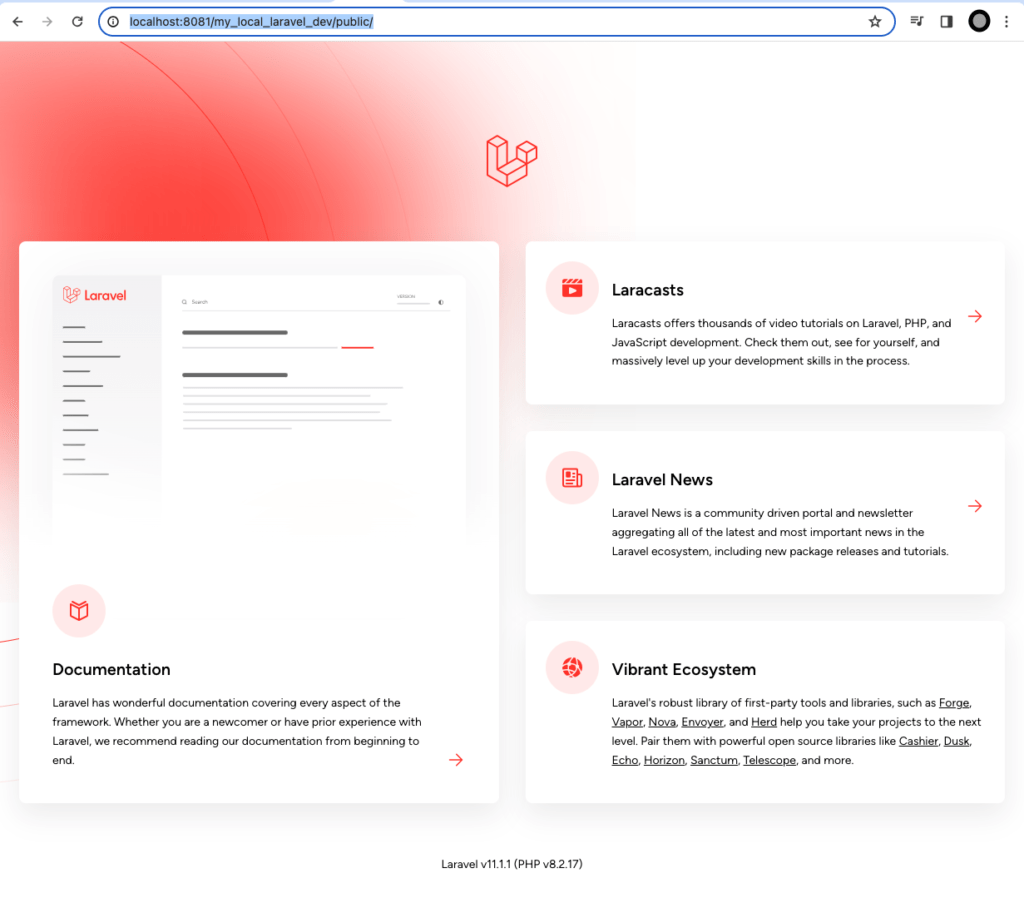
Hope you enjoyed and that this small application can make your life easier in starting your dev environment. Happy learning