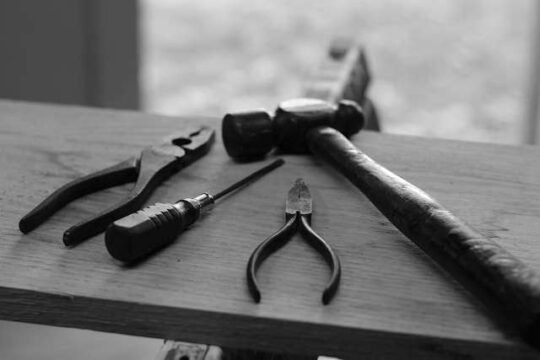
Introduction
Welcome to BrewedBrilliance.net, where we simplify complex tech topics for enthusiasts and professionals alike. Today, we’re diving into the world of SQL (Structured Query Language), the cornerstone of database management. Whether you’re new or not this SQL guide will provide an overview, from basic syntax to advanced usage, including tips on optimizing performance.
What is SQL?
SQL, or Structured Query Language, is a standardized language used to interact with relational databases. It enables users to create, read, update, and delete data, commonly referred to as CRUD operations. SQL is essential for managing and manipulating data stored in relational database management systems (RDBMS) like MySQL, PostgreSQL, SQL Server, and SQLite.
Basic SQL Syntax
Creating a Database
To create a new database, you use the CREATE DATABASE
statement:
CREATE DATABASE my_database;
Creating a Table
Once the database is created, you can create tables to store your data:
CREATE TABLE employees (
id INT PRIMARY KEY,
first_name VARCHAR(50),
last_name VARCHAR(50),
email VARCHAR(100),
department_id INT,
hire_date DATE
);
Inserting Data
To insert data into a table, use the INSERT INTO
statement:
INSERT INTO employees (id, first_name, last_name, email, department_id, hire_date)
VALUES (1, 'John', 'Doe', 'john.doe@example.com', 2, '2023-01-15');
Selecting Data
To retrieve data from a table, use the SELECT
statement:
# select all the employees
SELECT * FROM employees;
# Below examples for restricting the search
# OR, select where the name is John, will return all the employees which name is 'John'
SELECT * FROM employees where name="John";
# OR select all the employees that were hired after a certain date
SELECT * FROM employees where hire_date>"2022-01-01";
# OR we can combine those and select each "John" hired after a certain date
SELECT * FROM employees where name="John" and hire_date>"2022-01-01";
# OR we can select all the employees working for a specific department that were hired after a certain date
SELECT * FROM employees where name="John" and hire_date>"2022-01-01";
# OR we can do one OR the other
SELECT * FROM employees WHERE department_id=3 and hire_date>"2022-01-01";
# OR we can do one OR the other
SELECT * FROM employees where name="John" OR hire_date>"2022-01-01";
All the table fields can be used in all logical combinations.
We can also sort the result by adding the “ORDER BY” at the end of the select statement.
# select all the employees and sort by name
SELECT * FROM employees ORDER BY name ASC;
SELECT * FROM employees ORDER BY name DESC;
SELECT * FROM employees ORDER BY hire_date ASC;
SELECT * FROM employees ORDER BY hire_date DESC;
# and so on
Updating Data
To update existing data, use the UPDATE
statement:
UPDATE employees
SET email = 'john.newemail@example.com'
WHERE id = 1;
Deleting Data
To delete data from a table, use the DELETE
statement:
DELETE FROM employees
WHERE id = 1;
It is important to understand that the UPDATE and DELETE will apply that operation (update that column or delete that row) to ALL the records that are matching the WHERE clause, so it is important to understand that
DELETE FROM employees WHERE name='John';
Can have an unexpected result because will delete all those records where the name is John. It can be more than on
Advanced SQL Usage
SQL joins are used to combine rows from two or more tables based on a related column between them. They are fundamental in SQL for querying data that is spread across multiple tables in a relational database. Here’s a deeper look at the purpose and types of SQL joins:
Purpose of SQL Joins
- Data Retrieval: Joins allow you to retrieve related data from multiple tables in a single query, making it easier to compile comprehensive datasets.
- Data Analysis: By combining tables, you can perform complex analyses that would be difficult with a single table, such as understanding relationships between different entities.
- Data Integrity: Joins help maintain data integrity by ensuring that data spread across tables can be consistently queried and related.
Types of SQL Joins
For the next example we will need to create also a secondary table (departments)
CREATE TABLE departments (
id INT PRIMARY KEY,
department_name VARCHAR(100) NOT NULL
);
See the diagram below as reference for the next examples
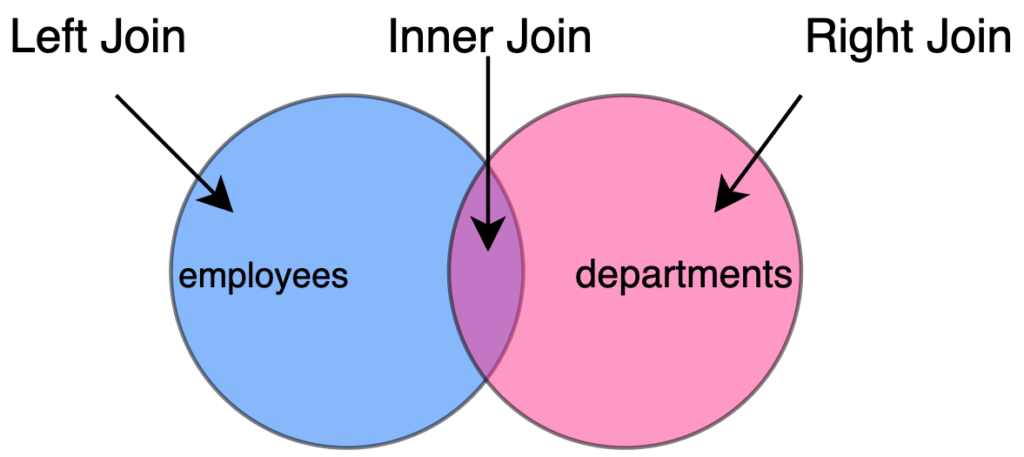
1. Inner Join
Usage: Fetches records that have matching values in both tables. It returns only the rows where there is a match in both tables.
Example:
Consider two tables, employees
and departments
.
SELECT employees.first_name, employees.last_name, departments.department_name
FROM employees
INNER JOIN departments ON employees.department_id = departments.id;
Result: This query returns the first name, last name, and department name of employees who are assigned to a department.
2. Left Join (Left Outer Join)
Usage: Returns all records from the left table and the matched records from the right table. If no match is found, NULL values are returned for columns from the right table.
Example:
SELECT employees.first_name, employees.last_name, departments.department_name
FROM employees
LEFT JOIN departments ON employees.department_id = departments.id;
Result: This query returns all employees, including those who are not assigned to any department. For employees without a department, the department_name will be NULL.
3. Right Join (Right Outer Join)
Usage: Returns all records from the right table and the matched records from the left table. If no match is found, NULL values are returned for columns from the left table.
Example:
SELECT employees.first_name, employees.last_name, departments.department_name
FROM employees
RIGHT JOIN departments ON employees.department_id = departments.id;
Result: This query returns all departments, including those that have no employees. For departments without employees, the first_name and last_name fields will be NULL.
4. Full Outer Join
Usage: Returns all records when there is a match in either left or right table records. If no match is found, NULL values are returned for columns from the table without a match.
Example:
SELECT employees.first_name, employees.last_name, departments.department_name
FROM employees
FULL OUTER JOIN departments ON employees.department_id = departments.id;
Result: This query returns all employees and all departments. For employees without a department, department_name will be NULL, and for departments without employees, first_name and last_name will be NULL.
Improving Performance with Indexes
Indexes improve the speed of data retrieval operations on a database table at the cost of additional space and slower performance on data modification operations. We will try to write a dedicated article on this topic but for now you can take the below examples as reference
Creating an Index
CREATE INDEX idx_last_name ON employees(last_name);
Identifying Indexes
You can check for existing indexes using the following query:
SHOW INDEX FROM employees;
Performance Considerations
- Index Selectivity: High selectivity indexes (unique or nearly unique) improve performance more significantly.
- Avoid Over-Indexing: Too many indexes can slow down data modification operations.
- Composite Indexes: Use composite indexes for queries involving multiple columns to improve query performance.
Summary
SQL is a powerful tool for managing and manipulating data in relational databases. Understanding basic syntax, such as creating databases and tables, as well as performing CRUD operations, is essential. Advanced concepts like joins and indexes are critical for efficient database management and performance optimization. By mastering these concepts, you can effectively manage large datasets and enhance the performance of your database applications.
Stay tuned to Brewed Brilliance for more insightful tech guides and tips!
Share this content: